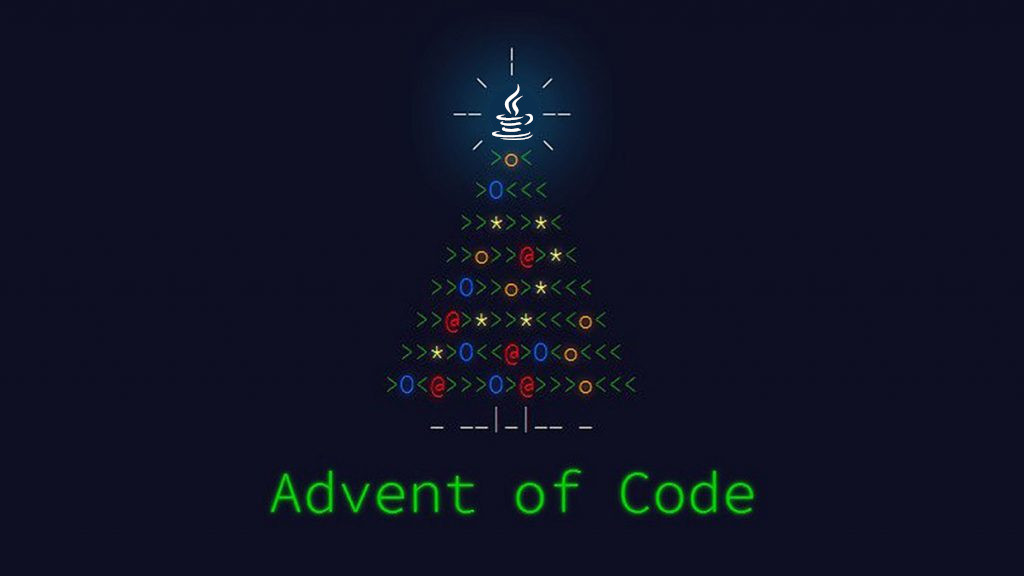
Advent of Code – Day 7: No Space Left On Device
Galician
O enunciado.
Vale, o enunciado está en inglés, e é unha matada traducilo. Así que podedes velo aquí en inglés. Nun futuro, se teño tempo, tradúzoo.
A solución.
Tiven que crear e usar varias clases (unha para o programa principal, dous clases, e DataInput). Tamén hai cambios no pom (para engadir Lombok) e no .gitignore.
Así que é un lío pegalo aquí. A solución pódese ver neste commit do meu repo de github: https://github.com/carlos-puente/advent-of-code-2022/commit/0c9f657e9c8045e64df4f0ff552f99622cd822f6
Regresar á páxina principal do Advent of Code 2022.
English
The statement
https://adventofcode.com/2022/day/7
Solution.
I had to create and use several classes (one for the main program, two classes, and DataInput). There are also changes in the pom (to adding Lombok) and in the .gitignore.
So it’s a mess to paste all here. The solution can be seen in this commit from my github repo:
https://github.com/carlos-puente/advent-of-code-2022/commit/0c9f657e9c8045e64df4f0ff552f99622cd822f6
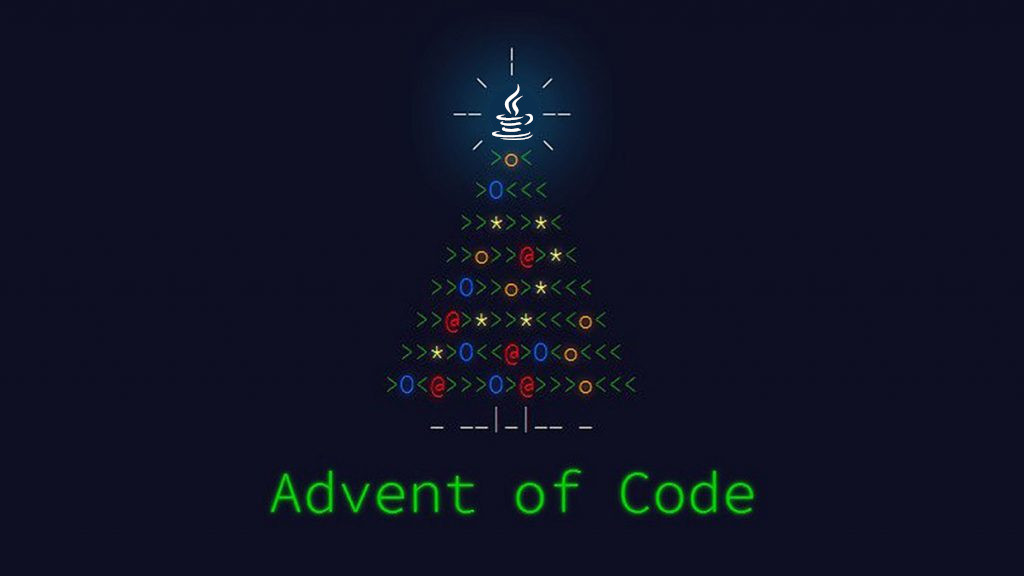
Advent of Code – Day 6: Tuning Trouble
Galician
O enunciado.
Vale, o enunciado está en inglés, e é unha matada traducilo. Así que podedes velo aquí en inglés. Nun futuro, se teño tempo, tradúzoo.
A solución.
package adventofcode2022;
import static data.DataInput.*;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class AdventOfCode6 {
public static void main(final String[] args) {
String streamBuffer = getDataStreamBuffer();
int part1Solution = getSolution(streamBuffer, 4);
int part2Solution = getSolution(streamBuffer, 14);
System.out.println("part 1 solution: " + part1Solution);
System.out.println("part 2 solution: " + part2Solution);
}
private static int getSolution(String streamBuffer, int numberOfCharacters) {
char[] dataStreamBuffer = streamBuffer.toCharArray();
for (int i = 0; i < dataStreamBuffer.length; i++) {
try {
List<Character> list = new ArrayList<>();
for (int j = 0; j < numberOfCharacters; j++) {
list.add(dataStreamBuffer[i + j]);
}
Set<Character> set = new HashSet<>(list);
if (set.size() == numberOfCharacters) {
String sequence = list.toString().replaceAll("\\[|\\]", "").replaceAll(",", "").replaceAll(" ", "");
return streamBuffer.indexOf(sequence) + numberOfCharacters;
}
} catch (IndexOutOfBoundsException ex) {
System.out.println("finished: sequence not found");
break;
}
}
return -1;
}
}
package data;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DataInput {
static String readFile(String path) {
byte[] encoded = new byte[0];
try {
encoded = Files.readAllBytes(Paths.get(path));
} catch (IOException e) {
throw new RuntimeException(e);
}
return new String(encoded, Charset.defaultCharset());
}
public static String getDataStreamBuffer() {
return readFile("src/main/resources/inputDay6.txt");
}
}
Regresar á páxina principal do Advent of Code 2022.
English
The statement
https://adventofcode.com/2022/day/6
Solution.
package adventofcode2022;
import static data.DataInput.*;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class AdventOfCode6 {
public static void main(final String[] args) {
String streamBuffer = getDataStreamBuffer();
int part1Solution = getSolution(streamBuffer, 4);
int part2Solution = getSolution(streamBuffer, 14);
System.out.println("part 1 solution: " + part1Solution);
System.out.println("part 2 solution: " + part2Solution);
}
private static int getSolution(String streamBuffer, int numberOfCharacters) {
char[] dataStreamBuffer = streamBuffer.toCharArray();
for (int i = 0; i < dataStreamBuffer.length; i++) {
try {
List<Character> list = new ArrayList<>();
for (int j = 0; j < numberOfCharacters; j++) {
list.add(dataStreamBuffer[i + j]);
}
Set<Character> set = new HashSet<>(list);
if (set.size() == numberOfCharacters) {
String sequence = list.toString().replaceAll("\\[|\\]", "").replaceAll(",", "").replaceAll(" ", "");
return streamBuffer.indexOf(sequence) + numberOfCharacters;
}
} catch (IndexOutOfBoundsException ex) {
System.out.println("finished: sequence not found");
break;
}
}
return -1;
}
}
package data;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DataInput {
static String readFile(String path) {
byte[] encoded = new byte[0];
try {
encoded = Files.readAllBytes(Paths.get(path));
} catch (IOException e) {
throw new RuntimeException(e);
}
return new String(encoded, Charset.defaultCharset());
}
public static String getDataStreamBuffer() {
return readFile("src/main/resources/inputDay6.txt");
}
}
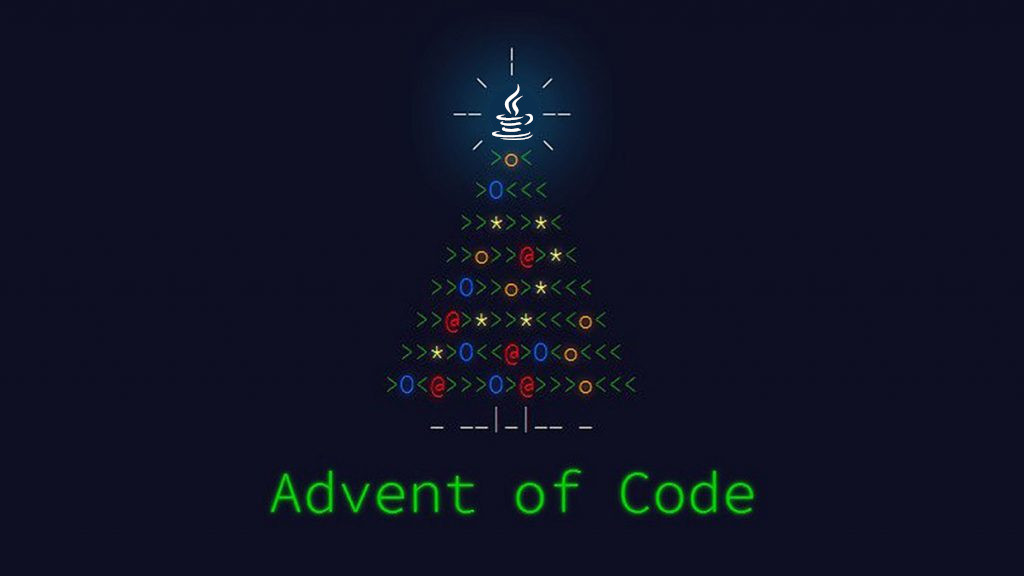
Advent of Code – Day 5: Supply Stacks
Galician
O enunciado.
Vale, o enunciado está en inglés, e é unha matada traducilo. Así que podedes velo aquí en inglés. Nun futuro, se teño tempo, tradúzoo.
A solución.
package adventofcode2022;
import static data.DataInput.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class AdventOfCode5 {
public static void main(final String[] args) {
final List<String> movements = getMovements();
List<List<String>> stacks = new ArrayList<>(getStacks());
List<List<String>> stacks2 = new ArrayList<>(getStacks());
for (final String movement : movements) {
final String[] splittedMovements = movement.split(",");
moveStacks(
stacks,
Integer.valueOf(splittedMovements[0]),
Integer.valueOf(splittedMovements[1]) - 1,
Integer.valueOf(splittedMovements[2]) - 1
);
moveStacksFull(
stacks2,
Integer.valueOf(splittedMovements[0]),
Integer.valueOf(splittedMovements[1]) - 1,
Integer.valueOf(splittedMovements[2]) - 1
);
}
System.out.println(showTopStaks(stacks));
System.out.println(showTopStaks(stacks2));
}
private static String showTopStaks(List<List<String>> stacks) {
String tops = "";
for (List<String> stack : stacks) {
tops += stack.get(0);
}
return tops;
}
private static void moveStacks(List<List<String>> stacks, final Integer elementsToMove, final Integer from, final Integer to) {
for (int i = 0; i < elementsToMove; i++) {
final String element = stacks.get(from).get(0);
stacks.get(from).remove(0);
Collections.reverse(stacks.get(to));
stacks.get(to).add(element);
Collections.reverse(stacks.get(to));
}
}
private static void moveStacksFull(List<List<String>> stacks, final Integer numElementsToMove, final Integer from, final Integer to) {
List<String> elementsToBeMoved = getStackElementsToMove(stacks, numElementsToMove, from);
Collections.reverse(elementsToBeMoved);
Collections.reverse(stacks.get(to));
stacks.get(to).addAll(elementsToBeMoved);
Collections.reverse(stacks.get(to));
}
private static List<String> getStackElementsToMove(List<List<String>> stacks, Integer numElementsToMove, Integer from) {
List<String> elementsToBeMoved = new ArrayList<>();
for (int i = 0; i < numElementsToMove; i++) {
elementsToBeMoved.add(stacks.get(from).get(0));
stacks.get(from).remove(0);
}
return elementsToBeMoved;
}
}
package data;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DataInput {
private static List<String> getListByPath(final String path) {
try {
return Files.readAllLines(Paths.get(path), Charset.defaultCharset());
} catch (final IOException e) {
throw new RuntimeException(e);
}
}
static List<String> stack1 = Stream.of("F", "H", "M", "T", "V", "L", "D").collect(Collectors.toList());
static List<String> stack2 = Stream.of("P", "N", "T", "C", "J", "G", "Q", "H").collect(Collectors.toList());
static List<String> stack3 = Stream.of("H", "P", "M", "D", "S", "R").collect(Collectors.toList());
static List<String> stack4 = Stream.of("F", "V", "B", "L").collect(Collectors.toList());
static List<String> stack5 = Stream.of("Q", "L", "G", "H", "N").collect(Collectors.toList());
static List<String> stack6 = Stream.of("P", "M", "R", "G", "D", "B", "W").collect(Collectors.toList());
static List<String> stack7 = Stream.of("Q", "L", "H", "C", "R", "N", "M", "G").collect(Collectors.toList());
static List<String> stack8 = Stream.of("W", "L", "C").collect(Collectors.toList());
static List<String> stack9 = Stream.of("T", "M", "Z", "J", "Q", "L", "D", "R").collect(Collectors.toList());
public static List<List<String>> getStacks() {
return new ArrayList<>(
Stream.of(new ArrayList<>(stack1), new ArrayList<>(stack2), new ArrayList<>(stack3), new ArrayList<>(stack4), new ArrayList<>(stack5),
new ArrayList<>(stack6), new ArrayList<>(stack7), new ArrayList<>(stack8), new ArrayList<>(stack9)).collect(Collectors.toList()));
}
public static List<String> getMovements() {
return getListByPath("src/main/resources/inputDay5.txt");
}
}
Regresar á páxina principal do Advent of Code 2022.
English
The statement
https://adventofcode.com/2022/day/5
Solution.
package adventofcode2022;
import static data.DataInput.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class AdventOfCode5 {
public static void main(final String[] args) {
final List<String> movements = getMovements();
List<List<String>> stacks = new ArrayList<>(getStacks());
List<List<String>> stacks2 = new ArrayList<>(getStacks());
for (final String movement : movements) {
final String[] splittedMovements = movement.split(",");
moveStacks(
stacks,
Integer.valueOf(splittedMovements[0]),
Integer.valueOf(splittedMovements[1]) - 1,
Integer.valueOf(splittedMovements[2]) - 1
);
moveStacksFull(
stacks2,
Integer.valueOf(splittedMovements[0]),
Integer.valueOf(splittedMovements[1]) - 1,
Integer.valueOf(splittedMovements[2]) - 1
);
}
System.out.println(showTopStaks(stacks));
System.out.println(showTopStaks(stacks2));
}
private static String showTopStaks(List<List<String>> stacks) {
String tops = "";
for (List<String> stack : stacks) {
tops += stack.get(0);
}
return tops;
}
private static void moveStacks(List<List<String>> stacks, final Integer elementsToMove, final Integer from, final Integer to) {
for (int i = 0; i < elementsToMove; i++) {
final String element = stacks.get(from).get(0);
stacks.get(from).remove(0);
Collections.reverse(stacks.get(to));
stacks.get(to).add(element);
Collections.reverse(stacks.get(to));
}
}
private static void moveStacksFull(List<List<String>> stacks, final Integer numElementsToMove, final Integer from, final Integer to) {
List<String> elementsToBeMoved = getStackElementsToMove(stacks, numElementsToMove, from);
Collections.reverse(elementsToBeMoved);
Collections.reverse(stacks.get(to));
stacks.get(to).addAll(elementsToBeMoved);
Collections.reverse(stacks.get(to));
}
private static List<String> getStackElementsToMove(List<List<String>> stacks, Integer numElementsToMove, Integer from) {
List<String> elementsToBeMoved = new ArrayList<>();
for (int i = 0; i < numElementsToMove; i++) {
elementsToBeMoved.add(stacks.get(from).get(0));
stacks.get(from).remove(0);
}
return elementsToBeMoved;
}
}
package data;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DataInput {
private static List<String> getListByPath(final String path) {
try {
return Files.readAllLines(Paths.get(path), Charset.defaultCharset());
} catch (final IOException e) {
throw new RuntimeException(e);
}
}
static List<String> stack1 = Stream.of("F", "H", "M", "T", "V", "L", "D").collect(Collectors.toList());
static List<String> stack2 = Stream.of("P", "N", "T", "C", "J", "G", "Q", "H").collect(Collectors.toList());
static List<String> stack3 = Stream.of("H", "P", "M", "D", "S", "R").collect(Collectors.toList());
static List<String> stack4 = Stream.of("F", "V", "B", "L").collect(Collectors.toList());
static List<String> stack5 = Stream.of("Q", "L", "G", "H", "N").collect(Collectors.toList());
static List<String> stack6 = Stream.of("P", "M", "R", "G", "D", "B", "W").collect(Collectors.toList());
static List<String> stack7 = Stream.of("Q", "L", "H", "C", "R", "N", "M", "G").collect(Collectors.toList());
static List<String> stack8 = Stream.of("W", "L", "C").collect(Collectors.toList());
static List<String> stack9 = Stream.of("T", "M", "Z", "J", "Q", "L", "D", "R").collect(Collectors.toList());
public static List<List<String>> getStacks() {
return new ArrayList<>(
Stream.of(new ArrayList<>(stack1), new ArrayList<>(stack2), new ArrayList<>(stack3), new ArrayList<>(stack4), new ArrayList<>(stack5),
new ArrayList<>(stack6), new ArrayList<>(stack7), new ArrayList<>(stack8), new ArrayList<>(stack9)).collect(Collectors.toList()));
}
public static List<String> getMovements() {
return getListByPath("src/main/resources/inputDay5.txt");
}
}
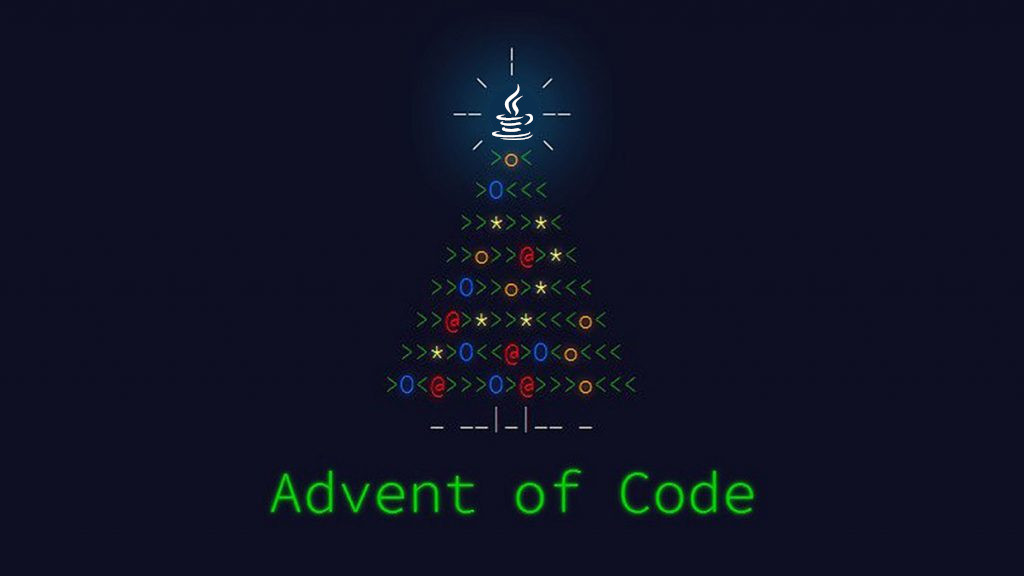
Advent of code – Day 4: Camp Cleanup
Galician
O enunciado.
Vale, o enunciado está en inglés, e é unha matada traducilo. Así que podedes velo aquí en inglés. Nun futuro, se teño tempo, tradúzoo.
A solución.
package adventofcode2022;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import data.DataInput;
public class AdventOfCode4 {
public static void main(final String[] args) {
final List<String> assignments = DataInput.getAssignmentPairs();
int overlaps = 0;
int partialOverlaps = 0;
for (final String assignmentsPair : assignments) {
final String[] pairs = assignmentsPair.split(",");
final String[] pair1 = pairs[0].split("-");
final String[] pair2 = pairs[1].split("-");
if (checkOverlapping(Integer.valueOf(pair1[0]), Integer.valueOf(pair1[1]), Integer.valueOf(pair2[0]), Integer.valueOf(pair2[1]))) {
overlaps++;
}
if (checkPartialOverlapping(Integer.valueOf(pair1[0]), Integer.valueOf(pair1[1]), Integer.valueOf(pair2[0]), Integer.valueOf(pair2[1]))) {
partialOverlaps++;
}
}
System.out.println(overlaps);
System.out.println(partialOverlaps);
}
private static boolean checkOverlapping(final int firstSectionStart, final int firstSectionEnd, final int secondSectionStart,
final int secondSectionEnd) {
return (firstSectionStart <= secondSectionStart && firstSectionEnd >= secondSectionEnd) ||
(secondSectionStart <= firstSectionStart && secondSectionEnd >= firstSectionEnd);
}
private static boolean checkPartialOverlapping(final int firstSectionStart, final int firstSectionEnd, final int secondSectionStart,
final int secondSectionEnd) {
final List<Integer> section1Elements = IntStream.rangeClosed(firstSectionStart, firstSectionEnd)
.boxed().collect(Collectors.toList());
final List<Integer> section2Elements = IntStream.rangeClosed(secondSectionStart, secondSectionEnd)
.boxed().collect(Collectors.toList());
final List<Integer> result =
section1Elements.stream().filter(section1 ->
section2Elements.stream().anyMatch(section2 -> section1 == section2)).collect(Collectors.toList());
return !result.isEmpty();
}
}
package data;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DataInput {
private static List<String> getListByPath(final String path) {
try {
return Files.readAllLines(Paths.get(path), Charset.defaultCharset());
} catch (final IOException e) {
throw new RuntimeException(e);
}
}
public static List<String> getMovements() {
return getListByPath("src/main/resources/inputDay5.txt");
}
}
Regresar á páxina principal do Advent of Code 2022.
English
The statement
https://adventofcode.com/2022/day/4
Solution.
package adventofcode2022;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import data.DataInput;
public class AdventOfCode4 {
public static void main(final String[] args) {
final List<String> assignments = DataInput.getAssignmentPairs();
int overlaps = 0;
int partialOverlaps = 0;
for (final String assignmentsPair : assignments) {
final String[] pairs = assignmentsPair.split(",");
final String[] pair1 = pairs[0].split("-");
final String[] pair2 = pairs[1].split("-");
if (checkOverlapping(Integer.valueOf(pair1[0]), Integer.valueOf(pair1[1]), Integer.valueOf(pair2[0]), Integer.valueOf(pair2[1]))) {
overlaps++;
}
if (checkPartialOverlapping(Integer.valueOf(pair1[0]), Integer.valueOf(pair1[1]), Integer.valueOf(pair2[0]), Integer.valueOf(pair2[1]))) {
partialOverlaps++;
}
}
System.out.println(overlaps);
System.out.println(partialOverlaps);
}
private static boolean checkOverlapping(final int firstSectionStart, final int firstSectionEnd, final int secondSectionStart,
final int secondSectionEnd) {
return (firstSectionStart <= secondSectionStart && firstSectionEnd >= secondSectionEnd) ||
(secondSectionStart <= firstSectionStart && secondSectionEnd >= firstSectionEnd);
}
private static boolean checkPartialOverlapping(final int firstSectionStart, final int firstSectionEnd, final int secondSectionStart,
final int secondSectionEnd) {
final List<Integer> section1Elements = IntStream.rangeClosed(firstSectionStart, firstSectionEnd)
.boxed().collect(Collectors.toList());
final List<Integer> section2Elements = IntStream.rangeClosed(secondSectionStart, secondSectionEnd)
.boxed().collect(Collectors.toList());
final List<Integer> result =
section1Elements.stream().filter(section1 ->
section2Elements.stream().anyMatch(section2 -> section1 == section2)).collect(Collectors.toList());
return !result.isEmpty();
}
}
package data;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DataInput {
private static List<String> getListByPath(final String path) {
try {
return Files.readAllLines(Paths.get(path), Charset.defaultCharset());
} catch (final IOException e) {
throw new RuntimeException(e);
}
}
public static List<String> getMovements() {
return getListByPath("src/main/resources/inputDay5.txt");
}
}
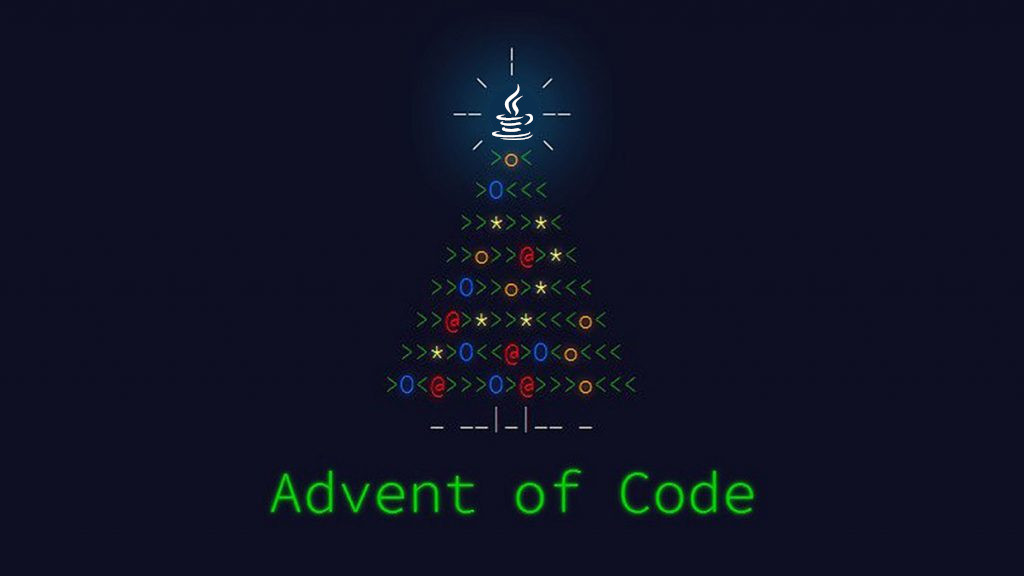
Advent of code – Day 3: Rucksack Reorganization
Galician
O enunciado.
Vale, o enunciado está en inglés, e é unha matada traducilo. Así que podedes velo en inglés. Nun futuro, se teño tempo, tradúzoo.
A solución utilizando os datos adaptados.
package adventofcode2022;
import static data.DataInput.*;
public class AdventOfCode3 {
public static void main(final String[] args) {
final String[] rucksacks = toArray(getRucksacks());
int points = 0;
for (final String rucksack : rucksacks) {
final int mid = rucksack.length() / 2; //get the middle of the String
final String[] parts = { rucksack.substring(0, mid), rucksack.substring(mid) };
points += getPointsByPriority(parts);
}
System.out.println(points);
points = 0;
final String[] groupThree = new String[] { "", "", "" };
int cont = 0;
for (int i = 0; i < rucksacks.length; i++) {
groupThree[cont++] = rucksacks[i];
if (cont % 3 == 0) {
points += getGroupPointsByPriority(groupThree);
cont = 0;
}
}
System.out.println(points);
}
private static int getGroupPointsByPriority(final String[] groupThree) {
final char common = '1';
for (int i = 0; i < groupThree[0].toCharArray().length; i++) {
for (int j = 0; j < groupThree[1].toCharArray().length; j++) {
if (groupThree[0].toCharArray()[i] == groupThree[1].toCharArray()[j]) {
for (int z = 0; z < groupThree[2].toCharArray().length; z++) {
if (groupThree[2].toCharArray()[z] == groupThree[0].toCharArray()[i]) {
return itemsValue.get(groupThree[2].toCharArray()[z]);
}
}
}
}
}
return 0;
}
private static int getPointsByPriority(final String[] parts) {
final char common = getCommonItem(parts[0], parts[1]);
if (common != '1') {
return itemsValue.get(common);
}
return 0;
}
private static char getCommonItem(final String part1, final String part2) {
for (final char part1char : part1.toCharArray()) {
for (final char part2char : part2.toCharArray()) {
if (part1char == part2char) {
return part1char;
}
}
}
return '1';
}
}
package data;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DataInput {
private static List<String> getListByPath(final String path) {
try {
return Files.readAllLines(Paths.get(path), Charset.defaultCharset());
} catch (final IOException e) {
throw new RuntimeException(e);
}
}
public static String[] toArray(final List<String> list) {
return list.toArray(String[]::new);
}
public static final Map<Character, Integer> itemsValue = new HashMap<>() {{
put('a', Integer.valueOf(1));
put('b', Integer.valueOf(2));
put('c', Integer.valueOf(3));
put('d', Integer.valueOf(4));
put('e', Integer.valueOf(5));
put('f', Integer.valueOf(6));
put('g', Integer.valueOf(7));
put('h', Integer.valueOf(8));
put('i', Integer.valueOf(9));
put('j', Integer.valueOf(10));
put('k', Integer.valueOf(11));
put('l', Integer.valueOf(12));
put('m', Integer.valueOf(13));
put('n', Integer.valueOf(14));
put('o', Integer.valueOf(17));
put('p', Integer.valueOf(16));
put('q', Integer.valueOf(17));
put('r', Integer.valueOf(18));
put('s', Integer.valueOf(19));
put('t', Integer.valueOf(20));
put('u', Integer.valueOf(21));
put('v', Integer.valueOf(22));
put('w', Integer.valueOf(23));
put('x', Integer.valueOf(24));
put('y', Integer.valueOf(25));
put('z', Integer.valueOf(26));
put('A', Integer.valueOf(27));
put('B', Integer.valueOf(28));
put('C', Integer.valueOf(29));
put('D', Integer.valueOf(30));
put('E', Integer.valueOf(31));
put('F', Integer.valueOf(32));
put('G', Integer.valueOf(33));
put('H', Integer.valueOf(34));
put('I', Integer.valueOf(35));
put('J', Integer.valueOf(36));
put('K', Integer.valueOf(37));
put('L', Integer.valueOf(38));
put('M', Integer.valueOf(39));
put('N', Integer.valueOf(40));
put('O', Integer.valueOf(41));
put('P', Integer.valueOf(42));
put('Q', Integer.valueOf(43));
put('R', Integer.valueOf(44));
put('S', Integer.valueOf(45));
put('T', Integer.valueOf(46));
put('U', Integer.valueOf(47));
put('V', Integer.valueOf(48));
put('W', Integer.valueOf(49));
put('X', Integer.valueOf(50));
put('Y', Integer.valueOf(51));
put('Z', Integer.valueOf(52));
}};
}
Regresar á páxina principal do Advent of Code 2022.
English
The statement
https://adventofcode.com/2022/day/3
Solution.
package adventofcode2022;
import static data.DataInput.*;
public class AdventOfCode3 {
public static void main(final String[] args) {
final String[] rucksacks = toArray(getRucksacks());
int points = 0;
for (final String rucksack : rucksacks) {
final int mid = rucksack.length() / 2; //get the middle of the String
final String[] parts = { rucksack.substring(0, mid), rucksack.substring(mid) };
points += getPointsByPriority(parts);
}
System.out.println(points);
points = 0;
final String[] groupThree = new String[] { "", "", "" };
int cont = 0;
for (int i = 0; i < rucksacks.length; i++) {
groupThree[cont++] = rucksacks[i];
if (cont % 3 == 0) {
points += getGroupPointsByPriority(groupThree);
cont = 0;
}
}
System.out.println(points);
}
private static int getGroupPointsByPriority(final String[] groupThree) {
final char common = '1';
for (int i = 0; i < groupThree[0].toCharArray().length; i++) {
for (int j = 0; j < groupThree[1].toCharArray().length; j++) {
if (groupThree[0].toCharArray()[i] == groupThree[1].toCharArray()[j]) {
for (int z = 0; z < groupThree[2].toCharArray().length; z++) {
if (groupThree[2].toCharArray()[z] == groupThree[0].toCharArray()[i]) {
return itemsValue.get(groupThree[2].toCharArray()[z]);
}
}
}
}
}
return 0;
}
private static int getPointsByPriority(final String[] parts) {
final char common = getCommonItem(parts[0], parts[1]);
if (common != '1') {
return itemsValue.get(common);
}
return 0;
}
private static char getCommonItem(final String part1, final String part2) {
for (final char part1char : part1.toCharArray()) {
for (final char part2char : part2.toCharArray()) {
if (part1char == part2char) {
return part1char;
}
}
}
return '1';
}
}
package data;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DataInput {
private static List<String> getListByPath(final String path) {
try {
return Files.readAllLines(Paths.get(path), Charset.defaultCharset());
} catch (final IOException e) {
throw new RuntimeException(e);
}
}
public static String[] toArray(final List<String> list) {
return list.toArray(String[]::new);
}
public static final Map<Character, Integer> itemsValue = new HashMap<>() {{
put('a', Integer.valueOf(1));
put('b', Integer.valueOf(2));
put('c', Integer.valueOf(3));
put('d', Integer.valueOf(4));
put('e', Integer.valueOf(5));
put('f', Integer.valueOf(6));
put('g', Integer.valueOf(7));
put('h', Integer.valueOf(8));
put('i', Integer.valueOf(9));
put('j', Integer.valueOf(10));
put('k', Integer.valueOf(11));
put('l', Integer.valueOf(12));
put('m', Integer.valueOf(13));
put('n', Integer.valueOf(14));
put('o', Integer.valueOf(17));
put('p', Integer.valueOf(16));
put('q', Integer.valueOf(17));
put('r', Integer.valueOf(18));
put('s', Integer.valueOf(19));
put('t', Integer.valueOf(20));
put('u', Integer.valueOf(21));
put('v', Integer.valueOf(22));
put('w', Integer.valueOf(23));
put('x', Integer.valueOf(24));
put('y', Integer.valueOf(25));
put('z', Integer.valueOf(26));
put('A', Integer.valueOf(27));
put('B', Integer.valueOf(28));
put('C', Integer.valueOf(29));
put('D', Integer.valueOf(30));
put('E', Integer.valueOf(31));
put('F', Integer.valueOf(32));
put('G', Integer.valueOf(33));
put('H', Integer.valueOf(34));
put('I', Integer.valueOf(35));
put('J', Integer.valueOf(36));
put('K', Integer.valueOf(37));
put('L', Integer.valueOf(38));
put('M', Integer.valueOf(39));
put('N', Integer.valueOf(40));
put('O', Integer.valueOf(41));
put('P', Integer.valueOf(42));
put('Q', Integer.valueOf(43));
put('R', Integer.valueOf(44));
put('S', Integer.valueOf(45));
put('T', Integer.valueOf(46));
put('U', Integer.valueOf(47));
put('V', Integer.valueOf(48));
put('W', Integer.valueOf(49));
put('X', Integer.valueOf(50));
put('Y', Integer.valueOf(51));
put('Z', Integer.valueOf(52));
}};
}
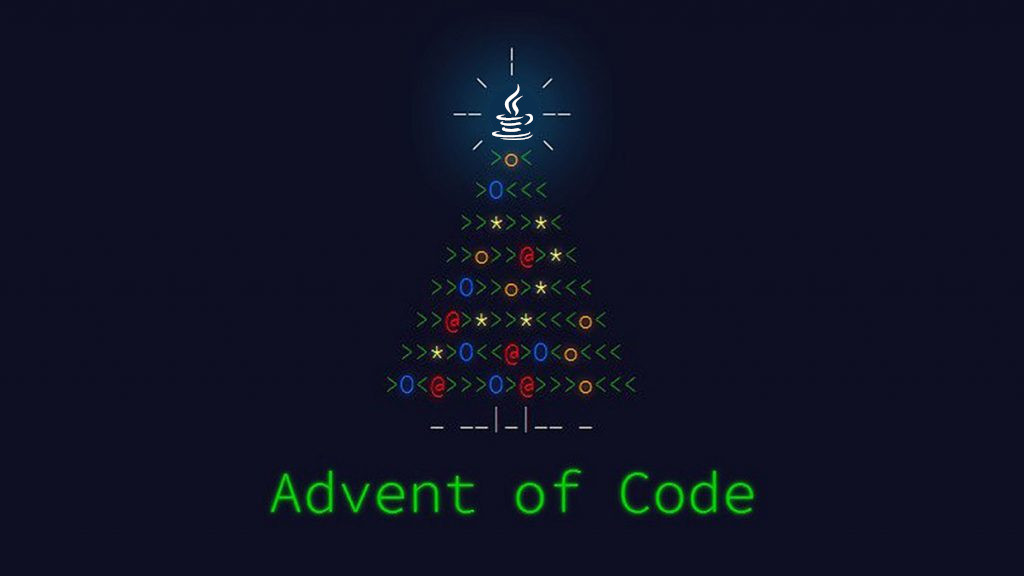
Advent of code – Day 2: Rock Paper Scissors
Galician
Parte un do problema.
O enunciado.
Vale, o enunciado está en inglés, e é unha matada traducilo. Así que podedes velo máis abaixo en inglés. Nun futuro, se teño tempo, tradúzoo.
Adaptando os datos de entrada.
Ok, utilizando o modo columna do Notepad++, e uns truquiños, convertín os datos de entrada en dous arrays de Strings, un para os nosos movementos, e outros para os movementos dos rivais.
A solución utilizando os datos adaptados.
Pois nada, que hai que xogar ao pedra, papel, tesoira. Temos os nosos movementos, e os do noso rival. Os puntos que vale cada xogada, e os puntos que nos dan polo movemento escollido. Só queda iterar e sumar.
import static data.DataInput.*;
import java.util.HashMap;
import java.util.Map;
public class AdventOfCode2 {
static final String ROCK = "A";
static final String PAPER = "B";
static final String OUR_ROCK = "X";
static final String OUR_PAPER = "Y";
static final String OUR_SCISSORS = "Z";
static final int WINING_POINTS = 6;
static final int DRAW_POINTS = 3;
static final Map<String, Integer> pointsBySelectedShape = new HashMap<>() {{
put(OUR_ROCK, Integer.valueOf(1));
put(OUR_PAPER, Integer.valueOf(2));
put(OUR_SCISSORS, Integer.valueOf(3));
}};
public static void main(final String[] args) {
//PART1
int points = 0;
for (int i = 0; i < theirMovements.length; i++) {
points = points + getRoundPoints(theirMovements[i], ourMovements[i]);
}
System.out.println(points);
}
/**
* @param theirMovement
* A for Rock, B for Paper, and C for Scissors
* @param ourMovement
* X for Rock, Y for Paper, and Z for Scissors
* @return points (1 for Rock, 2 for Paper, and 3 for Scissors) + (0 if you lost, 3 if the round was a draw, and 6 if you won).
*/
private static int getRoundPoints(final String theirMovement, final String ourMovement) {
final int points = pointsBySelectedShape.get(ourMovement);
if (ROCK.equalsIgnoreCase(theirMovement)) {
if (OUR_PAPER.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
} else if (OUR_SCISSORS.equalsIgnoreCase(ourMovement)) {
return points;
}
return points + DRAW_POINTS;
} else if (PAPER.equalsIgnoreCase(theirMovement)) {
if (OUR_ROCK.equalsIgnoreCase(ourMovement)) {
return points;
} else if (OUR_SCISSORS.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
}
return points + DRAW_POINTS;
} else {
if (OUR_ROCK.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
} else if (OUR_PAPER.equalsIgnoreCase(ourMovement)) {
return points;
}
return points + DRAW_POINTS;
}
}
}
}
Parte dous do problema.
O enunciado.
Vale, o enunciado está en inglés, e é unha matada traducilo. Así que podedes velo máis abaixo en inglés. Nun futuro, se teño tempo, tradúzoo.
A solución.
Temos que reutilizar os métodos anteriores, pero antes escollendo os movemenos que debemos facer, en base aos do rival, se queremos gañar, perder ou empatar. Os arrays están definidos en DataInput.
package adventofcode2022;
import static data.DataInput.*;
import java.util.HashMap;
import java.util.Map;
public class AdventOfCode2 {
static final String ROCK = "A";
static final String PAPER = "B";
static final String OUR_ROCK = "X";
static final String OUR_PAPER = "Y";
static final String OUR_SCISSORS = "Z";
static final String LOSE = "X";
static final String WIN = "Z";
static final int WINING_POINTS = 6;
static final int DRAW_POINTS = 3;
static final Map<String, Integer> pointsBySelectedShape = new HashMap<>() {{
put(OUR_ROCK, Integer.valueOf(1));
put(OUR_PAPER, Integer.valueOf(2));
put(OUR_SCISSORS, Integer.valueOf(3));
}};
public static void main(final String[] args) {
//PART1
int points = 0;
for (int i = 0; i < theirMovements.length; i++) {
points = points + getRoundPoints(theirMovements[i], ourMovements[i]);
}
System.out.println(points);
//PART 2
points = 0;
for (int i = 0; i < theirMovements.length; i++) {
points = points + getStrategyRoundPoints(theirMovements[i], ourMovements[i]);
}
System.out.println(points);
}
/**
* @param theirMovement
* A for Rock, B for Paper, and C for Scissors
* @param ourMovement
* X for Rock, Y for Paper, and Z for Scissors
* @return points (1 for Rock, 2 for Paper, and 3 for Scissors) + (0 if you lost, 3 if the round was a draw, and 6 if you won).
*/
private static int getRoundPoints(final String theirMovement, final String ourMovement) {
final int points = pointsBySelectedShape.get(ourMovement);
if (ROCK.equalsIgnoreCase(theirMovement)) {
if (OUR_PAPER.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
} else if (OUR_SCISSORS.equalsIgnoreCase(ourMovement)) {
return points;
}
return points + DRAW_POINTS;
} else if (PAPER.equalsIgnoreCase(theirMovement)) {
if (OUR_ROCK.equalsIgnoreCase(ourMovement)) {
return points;
} else if (OUR_SCISSORS.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
}
return points + DRAW_POINTS;
} else {
if (OUR_ROCK.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
} else if (OUR_PAPER.equalsIgnoreCase(ourMovement)) {
return points;
}
return points + DRAW_POINTS;
}
}
/**
* Obtains the shape based in our strategy, and gets the points for this round.
*
* @param theirMovement
* A for Rock, B for Paper, and C for Scissors
* @param ourDecission
* X lose, Y draw, and Z win
* @return points (1 for Rock, 2 for Paper, and 3 for Scissors) + (0 if you lost, 3 if the round was a draw, and 6 if you won).
*/
private static int getStrategyRoundPoints(final String theirMovement, final String ourDecission) {
final String shape = getShapeByStrategy(theirMovement, ourDecission);
return getRoundPoints(theirMovement, shape);
}
/**
* Returns the shape needed to lose, draw or win based in the movement of our rival.
*
* @param theirMovement
* A for Rock, B for Paper, and C for Scissors
* @param ourDecission
* X lose, Y draw, and Z win
* @return X for Rock, Y for Paper, and Z for Scissors
*/
private static String getShapeByStrategy(final String theirMovement, final String ourDecission) {
if (ROCK.equalsIgnoreCase(theirMovement)) {
if (LOSE.equalsIgnoreCase(ourDecission)) {
return OUR_SCISSORS;
} else if (WIN.equalsIgnoreCase(ourDecission)) {
return OUR_PAPER;
}
return OUR_ROCK;
} else if (PAPER.equalsIgnoreCase(theirMovement)) {
if (LOSE.equalsIgnoreCase(ourDecission)) {
return OUR_ROCK;
} else if (WIN.equalsIgnoreCase(ourDecission)) {
return OUR_SCISSORS;
}
return OUR_PAPER;
} else {
if (LOSE.equalsIgnoreCase(ourDecission)) {
return OUR_PAPER;
} else if (WIN.equalsIgnoreCase(ourDecission)) {
return OUR_ROCK;
}
return OUR_SCISSORS;
}
}
}
Regresar á páxina principal do Advent of Code 2022.
English
Part 1 of the problem.
The statement
— Day 2: Rock Paper Scissors —
The Elves begin to set up camp on the beach. To decide whose tent gets to be closest to the snack storage, a giant Rock Paper Scissors tournament is already in progress.
Rock Paper Scissors is a game between two players. Each game contains many rounds; in each round, the players each simultaneously choose one of Rock, Paper, or Scissors using a hand shape. Then, a winner for that round is selected: Rock defeats Scissors, Scissors defeats Paper, and Paper defeats Rock. If both players choose the same shape, the round instead ends in a draw.
Appreciative of your help yesterday, one Elf gives you an encrypted strategy guide (your puzzle input) that they say will be sure to help you win. “The first column is what your opponent is going to play:
A
for Rock,B
for Paper, andC
for Scissors. The second column–” Suddenly, the Elf is called away to help with someone’s tent.The second column, you reason, must be what you should play in response:
X
for Rock,Y
for Paper, andZ
for Scissors. Winning every time would be suspicious, so the responses must have been carefully chosen.The winner of the whole tournament is the player with the highest score. Your total score is the sum of your scores for each round. The score for a single round is the score for the shape you selected (1 for Rock, 2 for Paper, and 3 for Scissors) plus the score for the outcome of the round (0 if you lost, 3 if the round was a draw, and 6 if you won).
Since you can’t be sure if the Elf is trying to help you or trick you, you should calculate the score you would get if you were to follow the strategy guide.
For example, suppose you were given the following strategy guide:
A Y
B X
C Z
This strategy guide predicts and recommends the following:
In the first round, your opponent will choose Rock (
A
), and you should choose Paper (Y
). This ends in a win for you with a score of 8 (2 because you chose Paper + 6 because you won).In the second round, your opponent will choose Paper (
B
), and you should choose Rock (X
). This ends in a loss for you with a score of 1 (1 + 0).-The third round is a draw with both players choosing Scissors, giving you a score of 3 + 3 = 6.
In this example, if you were to follow the strategy guide, you would get a total score of
15
(8 + 1 + 6).What would your total score be if everything goes exactly according to your strategy guide?
Adapting the provided input data
Ok, using some Notepad++ column mode ,I splitted the input data in two columns, and with some tricks, I transform each column into a String array.
Solution using the adapted input.
Ok, we have to play Rock, paper, scissor. So we have their movements, and our movements. And the points of each movement, and the points for winning, losing and drawing. Note: in DataInput, I have declared each array.
import static data.DataInput.*;
import java.util.HashMap;
import java.util.Map;
public class AdventOfCode2 {
static final String ROCK = "A";
static final String PAPER = "B";
static final String OUR_ROCK = "X";
static final String OUR_PAPER = "Y";
static final String OUR_SCISSORS = "Z";
static final int WINING_POINTS = 6;
static final int DRAW_POINTS = 3;
static final Map<String, Integer> pointsBySelectedShape = new HashMap<>() {{
put(OUR_ROCK, Integer.valueOf(1));
put(OUR_PAPER, Integer.valueOf(2));
put(OUR_SCISSORS, Integer.valueOf(3));
}};
public static void main(final String[] args) {
//PART1
int points = 0;
for (int i = 0; i < theirMovements.length; i++) {
points = points + getRoundPoints(theirMovements[i], ourMovements[i]);
}
System.out.println(points);
}
/**
* @param theirMovement
* A for Rock, B for Paper, and C for Scissors
* @param ourMovement
* X for Rock, Y for Paper, and Z for Scissors
* @return points (1 for Rock, 2 for Paper, and 3 for Scissors) + (0 if you lost, 3 if the round was a draw, and 6 if you won).
*/
private static int getRoundPoints(final String theirMovement, final String ourMovement) {
final int points = pointsBySelectedShape.get(ourMovement);
if (ROCK.equalsIgnoreCase(theirMovement)) {
if (OUR_PAPER.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
} else if (OUR_SCISSORS.equalsIgnoreCase(ourMovement)) {
return points;
}
return points + DRAW_POINTS;
} else if (PAPER.equalsIgnoreCase(theirMovement)) {
if (OUR_ROCK.equalsIgnoreCase(ourMovement)) {
return points;
} else if (OUR_SCISSORS.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
}
return points + DRAW_POINTS;
} else {
if (OUR_ROCK.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
} else if (OUR_PAPER.equalsIgnoreCase(ourMovement)) {
return points;
}
return points + DRAW_POINTS;
}
}
}
}
Part 2 of the problem.
The statement.
The Elf finishes helping with the tent and sneaks back over to you. “Anyway, the second column says how the round needs to end: X
means you need to lose, Y
means you need to end the round in a draw, and Z
means you need to win. Good luck!”
The total score is still calculated in the same way, but now you need to figure out what shape to choose so the round ends as indicated. The example above now goes like this:
In the first round, your opponent will choose Rock (A
), and you need the round to end in a draw (Y
), so you also choose Rock. This gives you a score of 1 + 3 = 4.
In the second round, your opponent will choose Paper (B
), and you choose Rock so you lose (X
) with a score of 1 + 0 = 1.
In the third round, you will defeat your opponent’s Scissors with Rock for a score of 1 + 6 = 7.
Now that you’re correctly decrypting the ultra top secret strategy guide, you would get a total score of 12
.
Following the Elf’s instructions for the second column, what would your total score be if everything goes exactly according to your strategy guide?
Solution.
Ok we can reuse all the methods declared in part 1. But before that, we have to select the correct shape to lose, win or draw.
package adventofcode2022;
import static data.DataInput.*;
import java.util.HashMap;
import java.util.Map;
public class AdventOfCode2 {
static final String ROCK = "A";
static final String PAPER = "B";
static final String OUR_ROCK = "X";
static final String OUR_PAPER = "Y";
static final String OUR_SCISSORS = "Z";
static final String LOSE = "X";
static final String WIN = "Z";
static final int WINING_POINTS = 6;
static final int DRAW_POINTS = 3;
static final Map<String, Integer> pointsBySelectedShape = new HashMap<>() {{
put(OUR_ROCK, Integer.valueOf(1));
put(OUR_PAPER, Integer.valueOf(2));
put(OUR_SCISSORS, Integer.valueOf(3));
}};
public static void main(final String[] args) {
//PART1
int points = 0;
for (int i = 0; i < theirMovements.length; i++) {
points = points + getRoundPoints(theirMovements[i], ourMovements[i]);
}
System.out.println(points);
//PART 2
points = 0;
for (int i = 0; i < theirMovements.length; i++) {
points = points + getStrategyRoundPoints(theirMovements[i], ourMovements[i]);
}
System.out.println(points);
}
/**
* @param theirMovement
* A for Rock, B for Paper, and C for Scissors
* @param ourMovement
* X for Rock, Y for Paper, and Z for Scissors
* @return points (1 for Rock, 2 for Paper, and 3 for Scissors) + (0 if you lost, 3 if the round was a draw, and 6 if you won).
*/
private static int getRoundPoints(final String theirMovement, final String ourMovement) {
final int points = pointsBySelectedShape.get(ourMovement);
if (ROCK.equalsIgnoreCase(theirMovement)) {
if (OUR_PAPER.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
} else if (OUR_SCISSORS.equalsIgnoreCase(ourMovement)) {
return points;
}
return points + DRAW_POINTS;
} else if (PAPER.equalsIgnoreCase(theirMovement)) {
if (OUR_ROCK.equalsIgnoreCase(ourMovement)) {
return points;
} else if (OUR_SCISSORS.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
}
return points + DRAW_POINTS;
} else {
if (OUR_ROCK.equalsIgnoreCase(ourMovement)) {
return points + WINING_POINTS;
} else if (OUR_PAPER.equalsIgnoreCase(ourMovement)) {
return points;
}
return points + DRAW_POINTS;
}
}
/**
* Obtains the shape based in our strategy, and gets the points for this round.
*
* @param theirMovement
* A for Rock, B for Paper, and C for Scissors
* @param ourDecission
* X lose, Y draw, and Z win
* @return points (1 for Rock, 2 for Paper, and 3 for Scissors) + (0 if you lost, 3 if the round was a draw, and 6 if you won).
*/
private static int getStrategyRoundPoints(final String theirMovement, final String ourDecission) {
final String shape = getShapeByStrategy(theirMovement, ourDecission);
return getRoundPoints(theirMovement, shape);
}
/**
* Returns the shape needed to lose, draw or win based in the movement of our rival.
*
* @param theirMovement
* A for Rock, B for Paper, and C for Scissors
* @param ourDecission
* X lose, Y draw, and Z win
* @return X for Rock, Y for Paper, and Z for Scissors
*/
private static String getShapeByStrategy(final String theirMovement, final String ourDecission) {
if (ROCK.equalsIgnoreCase(theirMovement)) {
if (LOSE.equalsIgnoreCase(ourDecission)) {
return OUR_SCISSORS;
} else if (WIN.equalsIgnoreCase(ourDecission)) {
return OUR_PAPER;
}
return OUR_ROCK;
} else if (PAPER.equalsIgnoreCase(theirMovement)) {
if (LOSE.equalsIgnoreCase(ourDecission)) {
return OUR_ROCK;
} else if (WIN.equalsIgnoreCase(ourDecission)) {
return OUR_SCISSORS;
}
return OUR_PAPER;
} else {
if (LOSE.equalsIgnoreCase(ourDecission)) {
return OUR_PAPER;
} else if (WIN.equalsIgnoreCase(ourDecission)) {
return OUR_ROCK;
}
return OUR_SCISSORS;
}
}
}
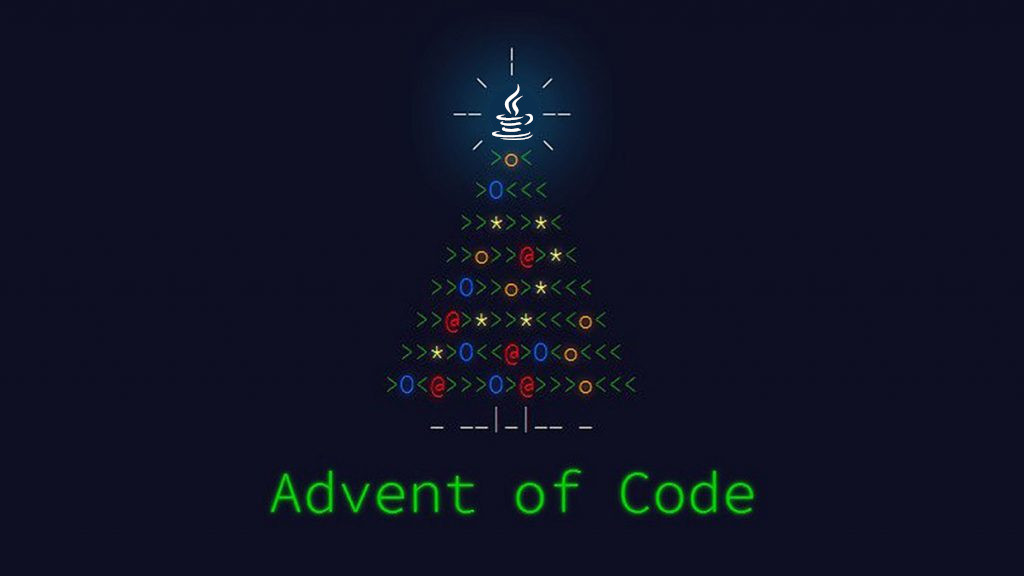
Advent of code – Day 1: Calorie Counting
Galician
Introducción.
Comezou o Advent of Code 2022! E ao igual que nas pasadas edicións, teremos que resolver dous puzzles, utilizando uns datos de entrada que nos proporcionarán xunto ao enunciado do problema.
Parte un do problema.
O enunciado.
Vale, o enunciado está en inglés, e é unha matada traducilo. Así que podedes velo, aquí. Nun futuro, se teño tempo, tradúzoo.
Adaptando os datos de entrada.
O enunciado do puzzle, proporciona unha colección única de datos, única por participante. Esta colección de datos (input), é moi longa, no meu caso, está formada por 2251 liñas (incluindo números e liñas baleiras). Pero nesta publicación, non utilizarei o meu input, se non o input de exemplo.
Despois de ler e comprender o enunciado, a forma máis rápida que ocurréuseme para utilizar este input, foi a de convertir cada liña nunha cadea de caracteres (String), e usalas como membros dun vector (array).
Como son moitas liñas, imos ver un truquiño no Notepad++, para facelo dunha forma moi rápida e sinxela.
Vamos, que o obxectivo é convertir esto:
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
a esto
"1000",
"2000",
"3000",
"",
"4000",
"",
"5000",
"6000",
"",
"7000",
"8000",
"9000",
"",
"10000"
E os pasos para conseguilo, son:
- Engadir ‘“‘ ao principio de cada liña:
- Acceder á sección substituír (reemplazar) en Notepad++ (Cntrl+H ou pulsar en Search -> Replace).
- Seleccionar a opción Regular Expression ao fondo da ventana.
- Escribir ^ no cadro de texto Find what.
- Escribir “ no cacro de texto Replace with.
- Colocar o cursor ao principio da primeira liña, para asegurar que todas as liñas serán cambiadas.
- Pulsar na opción Replace All.
- Engadir ‘“,‘ ao final de cada liña:
- Acceder á sección substituír (reemplazar) en Notepad++ (Cntrl+H ou pulsar en Search -> Replace).
- Seleccionar a opción Regular Expression ao fondo da ventana.
- Escribir $ no cadro de texto Find what.
- Escribir “ no cacro de texto Replace with.
- Colocar o cursor ao principio da primeira liña, para asegurar que todas as liñas serán cambiadas.
- Pulsar na opción Replace All.
- Eliminar a coma da última liña.
Como paso opcional, podemos unir todos os resultados nunha sola liña:
“1000”,”2000″,”3000″,””,”4000″,””,”5000″,”6000″,””,”7000″,”8000″,”9000″,””,”10000″ |
- Acceder á sección substituír (reemplazar) en Notepad++ (Cntrl+H ou pulsar en Search -> Replace).
- Seleccionar a opción Regular Expression ao fondo da ventana.
- Escribir \R no cadro de texto Find what.
- Deixar baleiro o cadro de texto Replace with.
- Colocar o cursor ao principio da primeira liña, para asegurar que todas as liñas serán cambiadas.
- Pulsar na opción Replace All.
A solución utilizando os datos adaptados.
Agora, podemos crear un array de Strings có input recén adaptado:
final String[] calories =new String[] {
"1000","2000","3000","","4000","","5000","6000","","7000","8000","9000","","10000"
}
E podémolo iterar para resolver a primeira parte do puzzle;:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class AdventCode1 {
public static void main(String[] args) {
final String[] calories = new String[] { INPUT_VALUES_HERE };
long sumCalories = 0;
List<Long> elves = new ArrayList<>();
for (String calory : calories) {
if (!calory.isEmpty()) {
//incrementar a suma de calorias ata que atopamos un espazo baleiro
sumCalories = sumCalories + Long.valueOf(calory);
} else {
//se atopamos o espazo baleiro, engadimos a suma
//e reiniciamos a variable para as sumas seguintes.
elves.add(sumCalories);
sumCalories = 0;
}
}
System.out.println(
"O elfo con máis calorías está a carrexar: " +
Collections.max(elves) + " calorías"
);
}
}
Parte dous do problema.
O enunciado.
O mesmiño que na parte 1, o enunciado está en inglés, e é unha matada traducilo. Así que podedes velo, aquí. Nun futuro, se teño tempo, tradúzoo.
A solución utilizando sort, sublist e stream.
Ok, temos a lista de calorías creada no paso 1. Polo que para atopar o total de calorías carrexado polos 3 elfos que máis calorías están a carrexar, podemos ordear a lista de forma descendente, crear unha sublista cós 3 primeiros elementos, e por último, sumalos usando streams.
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class AdventCode1 {
public static void main(String[] args) {
//PARTE 1
final String[] calories = new String[] { INPUT_VALUES_HERE };
long sumCalories = 0;
List<Long> elves = new ArrayList<>();
for (String calory : calories) {
if (!calory.isEmpty()) {
//incrementar a suma de calorias ata que atopamos un espazo baleiro
sumCalories = sumCalories + Long.valueOf(calory);
} else {
//se atopamos o espazo baleiro, engadimos a suma
//e reiniciamos a variable para as sumas seguintes.
elves.add(sumCalories);
sumCalories = 0;
}
}
System.out.println(
"O elfo con máis calorías está a carrexar: " +
Collections.max(elves) + " calorías"
);
//PARTE 2
Collections.sort(elves, Collections.reverseOrder()); //Ordear desc
System.out.println(
"Os 3 elfos que carrexan máis calorías, carrexan: " +
elves.subList(0,3)
);
System.out.println(
"E en total están a carrexar: " +
elves.subList(0,3).stream().collect(
Collectors.summingLong(Long::longValue)
)
+ " calorías"
);
}
}
E listo 😉
Regresar á páxina principal do Advent of Code 2022.
English
Introduction
The Advent of code has started! And as in last editions, every day we will have to solve two puzzles, based on an “input” that the program will give us.
Part 1 of the problem.
The statement
First of all, the statement of the first part can be read below:
Santa’s reindeer typically eat regular reindeer food, but they need a lot of magical energy to deliver presents on Christmas. For that, their favorite snack is a special type of star fruit that only grows deep in the jungle. The Elves have brought you on their annual expedition to the grove where the fruit grows.
To supply enough magical energy, the expedition needs to retrieve a minimum of fifty stars by December 25th. Although the Elves assure you that the grove has plenty of fruit, you decide to grab any fruit you see along the way, just in case.
Collect stars by solving puzzles. Two puzzles will be made available on each day in the Advent calendar; the second puzzle is unlocked when you complete the first. Each puzzle grants one star. Good luck!
The jungle must be too overgrown and difficult to navigate in vehicles or access from the air; the Elves’ expedition traditionally goes on foot. As your boats approach land, the Elves begin taking inventory of their supplies. One important consideration is food – in particular, the number of Calories each Elf is carrying (your puzzle input).
The Elves take turns writing down the number of Calories contained by the various meals, snacks, rations, etc. that they’ve brought with them, one item per line. Each Elf separates their own inventory from the previous Elf’s inventory (if any) by a blank line.
For example, suppose the Elves finish writing their items’ Calories and end up with the following list:
1000 2000 3000 4000 5000 6000 7000 8000 9000 10000
This list represents the Calories of the food carried by five Elves:
* The first Elf is carrying food with
1000
,2000
, and3000
Calories, a total of6000
Calories.– The second Elf is carrying one food item with
4000
Calories.– The third Elf is carrying food with
5000
and6000
Calories, a total of11000
Calories.– The fourth Elf is carrying food with
7000
,8000
, and9000
Calories, a total of24000
Calories.– The fifth Elf is carrying one food item with
10000
Calories.In case the Elves get hungry and need extra snacks, they need to know which Elf to ask: they’d like to know how many Calories are being carried by the Elf carrying the most Calories. In the example above, this is
24000
(carried by the fourth Elf).Find the Elf carrying the most Calories. How many total Calories is that Elf carrying?
Day 1 – Advent of code
Adapting the provided input data
The puzzle’s statement will provide us a longest and unique input, with a group of numbers separated by an empty line. In my case, the input has 2251 lines (including numbers and empty lines). But in this post, I will not use my input, I will use the example input.
So, the quickest way I could think of to use this input (and the empty lines as a separator), was to convert each row to string, and use them as member of an array. But there are many lines, so we can use some tricks in Notepad++ to quickly transform it.
So, the goal, using the example input is to convert from:
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
to
"1000",
"2000",
"3000",
"",
"4000",
"",
"5000",
"6000",
"",
"7000",
"8000",
"9000",
"",
"10000"
And the steps to achieve it, are:
- Adding ‘“‘at the beginning of each line:
- Go to Replace in Notepad++ (Ctrl+H or in menu bar, click Search -> Replace).
- Choose the Regular Expression option at the bottom of the dialog.
- Type ^ in the Find what textbox.
- Type “ in the Replace with textbox.
- Place cursor in the first line of the file to ensure all lines are affected.
- Click Replace All button.
- Add ‘“,‘ at the end of each line:
- Go to Replace in Notepad++ (Ctrl+H or in menu bar, click Search -> Replace).
- Choose the Regular Expression option at the bottom of the dialog.
- Type $ in the Find what textbox.
- Type “, in the Replace with textbox.
- Place cursor in the first line of the file to ensure all lines are affected.
- Click Replace All button.
- Delete last comma in the last line.
As an optional step, we can join all results in one line:
“1000”,”2000″,”3000″,””,”4000″,””,”5000″,”6000″,””,”7000″,”8000″,”9000″,””,”10000″ |
- Go to Replace in Notepad++ (Ctrl+H or in menu bar, click Search -> Replace).
- Choose the Regular Expression option at the bottom of the dialog.
- Type \R in the Find what textbox.
- Leave empty the Replace with textbox.
- Place cursor in the first line of the file to ensure all lines are affected.
- Click Replace All button.
Solution using the adapted input.
So, now in our code we can create an String array with the formatted input:
final String[] calories =new String[] {
"1000","2000","3000","","4000","","5000","6000","","7000","8000","9000","","10000"
}
And now, we can iterate this string to solve the first part of the puzzle:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class AdventCode1 {
public static void main(String[] args) {
final String[] calories = new String[] { INPUT_VALUES_HERE };
long sumCalories = 0;
List<Long> elves = new ArrayList<>();
for (String calory : calories) {
if (!calory.isEmpty()) {
//increase the sum of calories until a separator is found
sumCalories = sumCalories + Long.valueOf(calory);
} else {
//if the separator is found, we add the sum of calories,
//and restart the sum.
elves.add(sumCalories);
sumCalories = 0;
}
}
System.out.println(
"The Elf carrying the most calories carries: " +
Collections.max(elves) + " calories"
);
}
}
Part 2 of the problem.
The statement.
So the first part is solved, and we can continue with the second part, that has the following statement:
By the time you calculate the answer to the Elves’ question, they’ve already realized that the Elf carrying the most Calories of food might eventually run out of snacks.
To avoid this unacceptable situation, the Elves would instead like to know the total Calories carried by the top three Elves carrying the most Calories. That way, even if one of those Elves runs out of snacks, they still have two backups.
In the example above, the top three Elves are the fourth Elf (with
24000
Calories), then the third Elf (with11000
Calories), then the fifth Elf (with10000
Calories). The sum of the Calories carried by these three elves is45000
.Find the top three Elves carrying the most Calories. How many Calories are those Elves carrying in total?
Day 1 – Advent of code
Solution using sort, sublist, and streams.
Ok, we have a calories list created in part one. So to find the total calories carried by the top three Elves, we can sort the list (descending), and after that, create a sublist of first 3 elements, and last, sum them using streams.
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class AdventCode1 {
public static void main(String[] args) {
//PART1
final String[] calories = new String[] { INPUT_VALUES_HERE };
long sumCalories = 0;
List<Long> elves = new ArrayList<>();
for (String calory : calories) {
if (!calory.isEmpty()) {
//increase the sum of calories until a separator is found
sumCalories = sumCalories + Long.valueOf(calory);
} else {
//if the separator is found, we add the sum of calories,
//and restart the sum.
elves.add(sumCalories);
sumCalories = 0;
}
}
System.out.println(
"The Elf carrying the most calories carries: " +
Collections.max(elves) + " calories"
);
//PART 2
Collections.sort(elves, Collections.reverseOrder()); //Order DESC
System.out.println(
"The Elves carrying the most calories are carrying: " +
elves.subList(0,3)
);
System.out.println(
"And in total they are carrying: " +
elves.subList(0,3).stream().collect(
Collectors.summingLong(Long::longValue)
)
+ " calories"
);
}
}
And solved 🙂
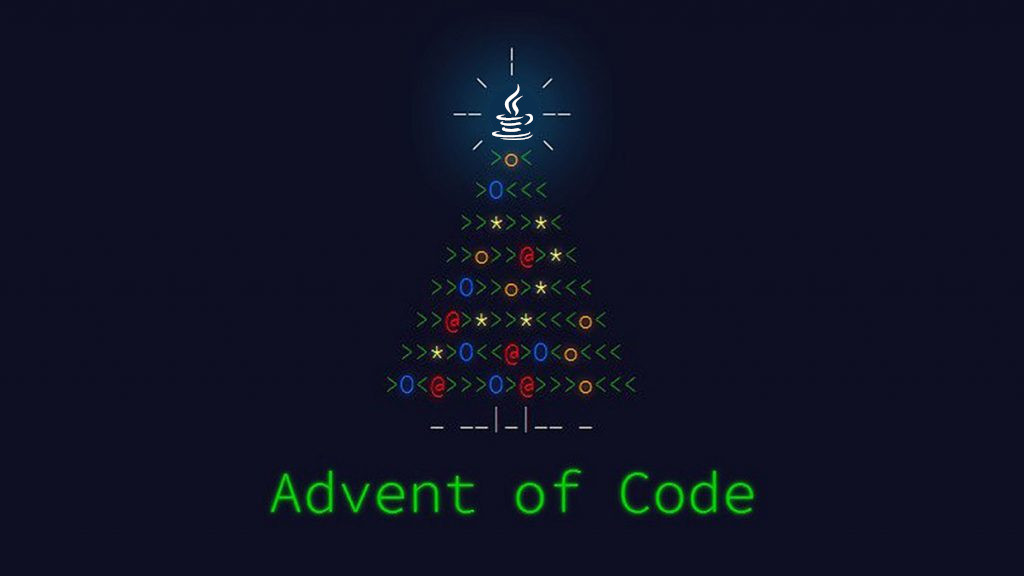
Advent of Code 2022.
Advent of Code is an Advent calendar of small programming puzzles for a variety of skill sets and skill levels that can be solved in any programming language you like. People use them as interview prep, company training, university coursework, practice problems, a speed contest, or to challenge each other.
Advent of Code – [More info]
Ok, this year I’ll try to do all the Advent of Code 2022 challenges using Java (if the algorithm allows me, if not, I’ll switch to python, hehe). I couldn’t complete it last year, because it was impossible for me to combine it with work and family life… but let’s see, hehe.
Below, I’ll publish a list of each daily challenge. And source code will be available in this github repo. Let’s have fun!
- Day 1: Calorie Counting. [Galician] [English]
- Day 2: Rock Paper Scissors [Galician] [English]
- Day 3: Rucksack Reorganization [Galician] [English]
- Day 4: Camp Cleanup [Galician] [English]
- Day 5: Supply Stacks [Galician] [English]
- Day 6: Tuning Trouble [Galician] [English]
- Day 7: No Space Left On Device [Galician] [English]
- Day 8: ?
- Day 9: ?
- Day 10: ?
- Day 11: ?
- Day 12: ?
- Day 13: ?
- Day 14: ?
- Day 15: ?
- Day 16: ?
- Day 17: ?
- Day 18: ?
- Day 19: ?
- Day 20: ?
- Day 21: ?
- Day 22: ?
- Day 23: ?
- Day 24: ?
- Day 25: ?
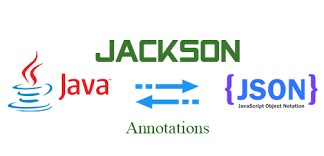
Tip: [Java / REST] Creating a Jackson multi date format deserializer.
In a microservice that is used by several portals, I have found the need that in certain endpoints, the dates received in the JSON of the requests can have different formats.
This is a problem since the Jackson @JsonFormat tag only allows us to declare a single pattern, like:
@JsonFormat(pattern = "yyyy-MM-dd")
private LocalDate incidentDate;
Luckily, there is another tag that allows us to carry out this development: @JsonDeserialize.
@JsonDeserialize(using = MultiDateDeserializer.class)
private LocalDate incidentDate;
A@JsonDeserialize allows us to specify a class that will perform custom deserialization. This is very useful in cases like this, where we need to perform additional steps in our requests.
package me.carlosjai.example.utils;
import java.io.IOException;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeParseException;
import java.util.Arrays;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import org.apache.commons.lang3.StringUtils;
import static me.carlosjai.example.utils.DateFormats.INTERNATIONAL_DATE_FORMATS;
public class MultiDateDeserializer extends StdDeserializer<LocalDate> {
private static final long serialVersionUID = 1L;
public MultiDateDeserializer() {
this(null);
}
public MultiDateDeserializer(Class<?> vc) {
super(vc);
}
@Override
/**
* Deserializes a custom date in several international date formats
*/
public LocalDate deserialize(JsonParser jp, DeserializationContext ctxt) throws IOException {
final String date =((JsonNode)jp.getCodec().readTree(jp)).textValue();
if(date== null || StringUtils.isBlank(date)) {
return null;
}
for (String dateFormat : INTERNATIONAL_DATE_FORMATS) {
try {
return LocalDate.parse(date, DateTimeFormatter.ofPattern(dateFormat));
} catch (DateTimeParseException e) {
}
}
throw new JsonParseException(jp, "Unparseable date: \"" + date + "\". Supported formats: " + Arrays.toString(INTERNATIONAL_DATE_FORMATS));
}
}
And the DateFormats class:
package me.carlosjai.example.utils;
public class DateFormats {
public static final String LITHUANIA_DATE_FORMAT = "yyyy-MM-dd";
public static final String LATVIA_DATE_FORMAT = "dd.MM.yyyy.";
public static final String[] INTERNATIONAL_DATE_FORMATS = new String[] {
LITHUANIA_DATE_FORMAT,
LATVIA_DATE_FORMAT,
};
}
And… Its done!
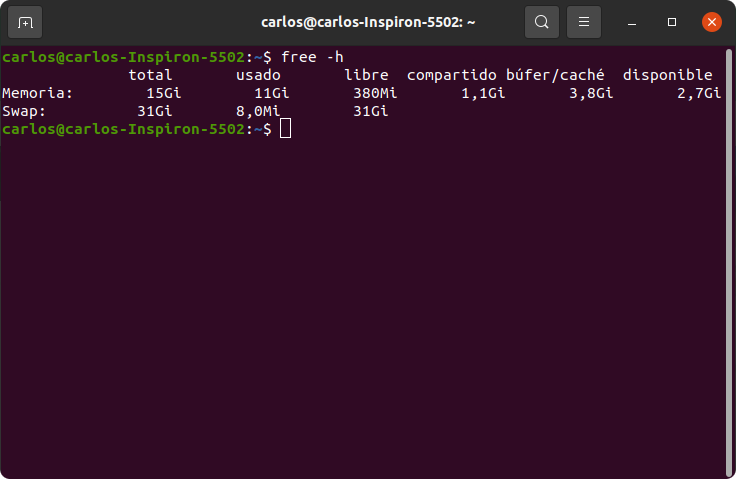
How to increase swap size in Ubuntu 20.04
I’m my actual job, I have a very powerful laptop. A Dell Inspiron 15 5000. This laptop was delivered to me the laptop with an Ubuntu distro installed, so I only had to setup all the environments.
Last month I had a lot of performance issues and the system was constantly freezing. First only with Slack notifications, but the last weeks, also with compilations of projects, or chrome tab loadings.
What was the reason for these performance problems? Shit! I only had 2GB of swap memory available. For a computer with 16 GB of RAM, the recomendations are the same amount of swap (1,5x or 2x if you want hibernation), so, I decided to increase my swap file size to 32GB. The steps to do it, are the next:
- We need to disable the swapping.
$ sudo sudo swapoff -a
- Next, we need to resize the swap file
$ sudo dd if=/dev/zero of=/swapfile bs=4G count=16
- Now, we have to change the swap file permissions
$ sudo chmod 600 /swapfile
- And convert it in swap
$ sudo mkswap /swapfile
- Activate it
$ sudo swapon /swapfile
- And add to /etc/fstab if not present
/swapfile none swap sw 0 0
And all my problems of perfomance are solved! You can see the available memory with:
$ sudo free -h